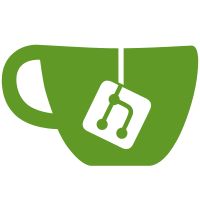
1. Rename in a backward compatible way 2. Remove gosec default exclude list because gosec is already disabled by default. 3. Warn about unmatched linter names in //nolint directives 4. Process linter names in //nolint directives in upper case 5. Disable gosec for golangci-lint in .golangci.yml
115 lines
3.4 KiB
Go
115 lines
3.4 KiB
Go
// Code generated by MockGen. DO NOT EDIT.
|
|
// Source: log.go
|
|
|
|
package logutils
|
|
|
|
import (
|
|
gomock "github.com/golang/mock/gomock"
|
|
reflect "reflect"
|
|
)
|
|
|
|
// MockLog is a mock of Log interface
|
|
type MockLog struct {
|
|
ctrl *gomock.Controller
|
|
recorder *MockLogMockRecorder
|
|
}
|
|
|
|
// MockLogMockRecorder is the mock recorder for MockLog
|
|
type MockLogMockRecorder struct {
|
|
mock *MockLog
|
|
}
|
|
|
|
// NewMockLog creates a new mock instance
|
|
func NewMockLog(ctrl *gomock.Controller) *MockLog {
|
|
mock := &MockLog{ctrl: ctrl}
|
|
mock.recorder = &MockLogMockRecorder{mock}
|
|
return mock
|
|
}
|
|
|
|
// EXPECT returns an object that allows the caller to indicate expected use
|
|
func (_m *MockLog) EXPECT() *MockLogMockRecorder {
|
|
return _m.recorder
|
|
}
|
|
|
|
// Fatalf mocks base method
|
|
func (_m *MockLog) Fatalf(format string, args ...interface{}) {
|
|
_s := []interface{}{format}
|
|
for _, _x := range args {
|
|
_s = append(_s, _x)
|
|
}
|
|
_m.ctrl.Call(_m, "Fatalf", _s...)
|
|
}
|
|
|
|
// Fatalf indicates an expected call of Fatalf
|
|
func (_mr *MockLogMockRecorder) Fatalf(arg0 interface{}, arg1 ...interface{}) *gomock.Call {
|
|
_s := append([]interface{}{arg0}, arg1...)
|
|
return _mr.mock.ctrl.RecordCallWithMethodType(_mr.mock, "Fatalf", reflect.TypeOf((*MockLog)(nil).Fatalf), _s...)
|
|
}
|
|
|
|
// Errorf mocks base method
|
|
func (_m *MockLog) Errorf(format string, args ...interface{}) {
|
|
_s := []interface{}{format}
|
|
for _, _x := range args {
|
|
_s = append(_s, _x)
|
|
}
|
|
_m.ctrl.Call(_m, "Errorf", _s...)
|
|
}
|
|
|
|
// Errorf indicates an expected call of Errorf
|
|
func (_mr *MockLogMockRecorder) Errorf(arg0 interface{}, arg1 ...interface{}) *gomock.Call {
|
|
_s := append([]interface{}{arg0}, arg1...)
|
|
return _mr.mock.ctrl.RecordCallWithMethodType(_mr.mock, "Errorf", reflect.TypeOf((*MockLog)(nil).Errorf), _s...)
|
|
}
|
|
|
|
// Warnf mocks base method
|
|
func (_m *MockLog) Warnf(format string, args ...interface{}) {
|
|
_s := []interface{}{format}
|
|
for _, _x := range args {
|
|
_s = append(_s, _x)
|
|
}
|
|
_m.ctrl.Call(_m, "Warnf", _s...)
|
|
}
|
|
|
|
// Warnf indicates an expected call of Warnf
|
|
func (_mr *MockLogMockRecorder) Warnf(arg0 interface{}, arg1 ...interface{}) *gomock.Call {
|
|
_s := append([]interface{}{arg0}, arg1...)
|
|
return _mr.mock.ctrl.RecordCallWithMethodType(_mr.mock, "Warnf", reflect.TypeOf((*MockLog)(nil).Warnf), _s...)
|
|
}
|
|
|
|
// Infof mocks base method
|
|
func (_m *MockLog) Infof(format string, args ...interface{}) {
|
|
_s := []interface{}{format}
|
|
for _, _x := range args {
|
|
_s = append(_s, _x)
|
|
}
|
|
_m.ctrl.Call(_m, "Infof", _s...)
|
|
}
|
|
|
|
// Infof indicates an expected call of Infof
|
|
func (_mr *MockLogMockRecorder) Infof(arg0 interface{}, arg1 ...interface{}) *gomock.Call {
|
|
_s := append([]interface{}{arg0}, arg1...)
|
|
return _mr.mock.ctrl.RecordCallWithMethodType(_mr.mock, "Infof", reflect.TypeOf((*MockLog)(nil).Infof), _s...)
|
|
}
|
|
|
|
// Child mocks base method
|
|
func (_m *MockLog) Child(name string) Log {
|
|
ret := _m.ctrl.Call(_m, "Child", name)
|
|
ret0, _ := ret[0].(Log)
|
|
return ret0
|
|
}
|
|
|
|
// Child indicates an expected call of Child
|
|
func (_mr *MockLogMockRecorder) Child(arg0 interface{}) *gomock.Call {
|
|
return _mr.mock.ctrl.RecordCallWithMethodType(_mr.mock, "Child", reflect.TypeOf((*MockLog)(nil).Child), arg0)
|
|
}
|
|
|
|
// SetLevel mocks base method
|
|
func (_m *MockLog) SetLevel(level LogLevel) {
|
|
_m.ctrl.Call(_m, "SetLevel", level)
|
|
}
|
|
|
|
// SetLevel indicates an expected call of SetLevel
|
|
func (_mr *MockLogMockRecorder) SetLevel(arg0 interface{}) *gomock.Call {
|
|
return _mr.mock.ctrl.RecordCallWithMethodType(_mr.mock, "SetLevel", reflect.TypeOf((*MockLog)(nil).SetLevel), arg0)
|
|
}
|