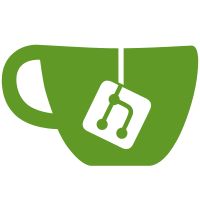
1. Rename in a backward compatible way 2. Remove gosec default exclude list because gosec is already disabled by default. 3. Warn about unmatched linter names in //nolint directives 4. Process linter names in //nolint directives in upper case 5. Disable gosec for golangci-lint in .golangci.yml
80 lines
1.5 KiB
Go
80 lines
1.5 KiB
Go
package linter
|
|
|
|
const (
|
|
PresetFormatting = "format"
|
|
PresetComplexity = "complexity"
|
|
PresetStyle = "style"
|
|
PresetBugs = "bugs"
|
|
PresetUnused = "unused"
|
|
PresetPerformance = "performance"
|
|
)
|
|
|
|
type Config struct {
|
|
Linter Linter
|
|
EnabledByDefault bool
|
|
DoesFullImport bool
|
|
NeedsSSARepr bool
|
|
InPresets []string
|
|
Speed int // more value means faster execution of linter
|
|
AlternativeNames []string
|
|
|
|
OriginalURL string // URL of original (not forked) repo, needed for autogenerated README
|
|
}
|
|
|
|
func (lc Config) WithFullImport() Config {
|
|
lc.DoesFullImport = true
|
|
return lc
|
|
}
|
|
|
|
func (lc Config) WithSSA() Config {
|
|
lc.DoesFullImport = true
|
|
lc.NeedsSSARepr = true
|
|
return lc
|
|
}
|
|
|
|
func (lc Config) WithPresets(presets ...string) Config {
|
|
lc.InPresets = presets
|
|
return lc
|
|
}
|
|
|
|
func (lc Config) WithSpeed(speed int) Config {
|
|
lc.Speed = speed
|
|
return lc
|
|
}
|
|
|
|
func (lc Config) WithURL(url string) Config {
|
|
lc.OriginalURL = url
|
|
return lc
|
|
}
|
|
|
|
func (lc Config) WithAlternativeNames(names ...string) Config {
|
|
lc.AlternativeNames = names
|
|
return lc
|
|
}
|
|
|
|
func (lc Config) NeedsProgramLoading() bool {
|
|
return lc.DoesFullImport
|
|
}
|
|
|
|
func (lc Config) NeedsSSARepresentation() bool {
|
|
return lc.NeedsSSARepr
|
|
}
|
|
|
|
func (lc Config) GetSpeed() int {
|
|
return lc.Speed
|
|
}
|
|
|
|
func (lc Config) AllNames() []string {
|
|
return append([]string{lc.Name()}, lc.AlternativeNames...)
|
|
}
|
|
|
|
func (lc Config) Name() string {
|
|
return lc.Linter.Name()
|
|
}
|
|
|
|
func NewConfig(linter Linter) *Config {
|
|
return &Config{
|
|
Linter: linter,
|
|
}
|
|
}
|