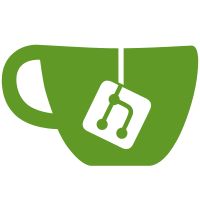
Also do following improvements: - show proper sublinter name for megacheck sublinters - refactor and make more simple and robust megacheck merging/optimizing - improve handling of unknown linter names in //nolint directives - minimize diff of our megacheck version from the upstream, https://github.com/golang/go/issues/29612 blocks usage of the upstream version - support the new `stylecheck` linter - improve tests coverage for megacheck and nolint related cases - update and use upstream versions of unparam and interfacer instead of forked ones - don't use golangci/tools repo anymore - fix newly found issues after updating linters Also should be noted that megacheck works much faster and consumes less memory in the newest release, therefore golangci-lint works noticeably faster and consumes less memory for large repos. Relates: #314
47 lines
937 B
Go
47 lines
937 B
Go
package golinters
|
|
|
|
import (
|
|
"context"
|
|
|
|
"mvdan.cc/unparam/check"
|
|
|
|
"github.com/golangci/golangci-lint/pkg/lint/linter"
|
|
"github.com/golangci/golangci-lint/pkg/result"
|
|
)
|
|
|
|
type Unparam struct{}
|
|
|
|
func (Unparam) Name() string {
|
|
return "unparam"
|
|
}
|
|
|
|
func (Unparam) Desc() string {
|
|
return "Reports unused function parameters"
|
|
}
|
|
|
|
func (lint Unparam) Run(ctx context.Context, lintCtx *linter.Context) ([]result.Issue, error) {
|
|
us := &lintCtx.Settings().Unparam
|
|
|
|
c := &check.Checker{}
|
|
c.CallgraphAlgorithm(us.Algo)
|
|
c.CheckExportedFuncs(us.CheckExported)
|
|
c.Packages(lintCtx.Packages)
|
|
c.ProgramSSA(lintCtx.SSAProgram)
|
|
|
|
unparamIssues, err := c.Check()
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
var res []result.Issue
|
|
for _, i := range unparamIssues {
|
|
res = append(res, result.Issue{
|
|
Pos: lintCtx.Program.Fset.Position(i.Pos()),
|
|
Text: markIdentifiers(i.Message()),
|
|
FromLinter: lint.Name(),
|
|
})
|
|
}
|
|
|
|
return res, nil
|
|
}
|